Getting Started with TypeScript
By Emily Clark • 3/10/2025
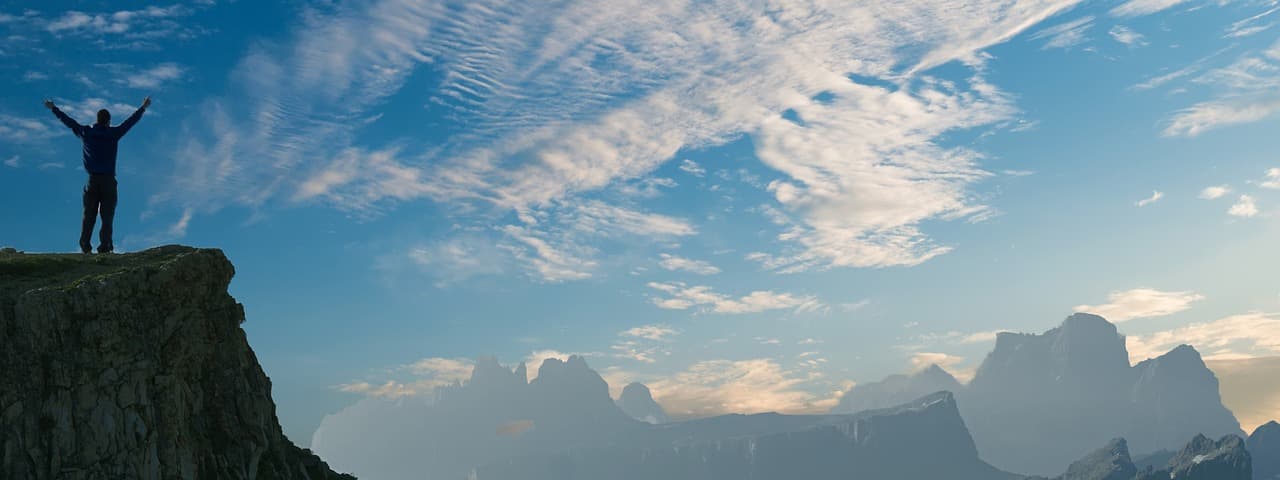
TypeScript is a strongly typed programming language that builds on JavaScript, giving you better tooling at any scale. It helps developers write safer and more maintainable code by catching errors early during development. By introducing static types, TypeScript allows developers to define the shape of objects, functions, and variables, reducing the likelihood of runtime errors.
One of the key benefits of TypeScript is its ability to provide a seamless development experience. With features like autocompletion, type checking, and advanced refactoring tools, TypeScript integrates smoothly with modern IDEs, making it easier to write and maintain complex applications. Additionally, TypeScript's compatibility with JavaScript ensures that you can gradually adopt it in existing projects without a complete rewrite.
In this guide, we will explore the basics of TypeScript, including its syntax, features, and how to integrate it into your projects. We will cover topics such as:
- Type Annotations: Learn how to define types for variables, function parameters, and return values to make your code more predictable.
- Interfaces and Types: Understand how to create reusable type definitions to enforce consistency across your codebase.
- Classes and Inheritance: Discover how TypeScript enhances object-oriented programming with features like access modifiers and abstract classes.
- Generics: Explore how to write flexible and reusable components using generics.
- Modules and Namespaces: Organize your code effectively with TypeScript's module system.
- Tooling and Configuration: Set up TypeScript in your project with tools like
tsconfig.json
and integrate it with popular build systems.
Here are some code examples to help you get started:
Type Annotations
// Defining types for variables
let username: string = \"JohnDoe\";
let age: number = 30;
let isActive: boolean = true;
// Function with type annotations
function greet(name: string): string {
return `Hello, ${name}!`;
}
console.log(greet(username));
Interfaces
// Defining an interface
interface User {
id: number;
name: string;
email: string;
}
// Using the interface
const user: User = {
id: 1,
name: \"Jane Doe\",
email: \"jane.doe@example.com\"
};
console.log(user);
Classes and Inheritance
// Defining a class
class Animal {
constructor(public name: string) {}
makeSound(): void {
console.log(\"Generic animal sound\");
}
}
// Inheriting from a class
class Dog extends Animal {
makeSound(): void {
console.log(\"Woof! Woof!\");
}
}
const dog = new Dog(\"Buddy\");
dog.makeSound();
Generics
// Generic function
function identity(value: T): T {
return value;
}
console.log(identity(\"Hello, TypeScript!\"));
console.log(identity(42));
Whether you're a beginner or an experienced developer, TypeScript can enhance your productivity and code quality. By the end of this guide, you'll have a solid understanding of TypeScript's core concepts and be ready to apply them to your own projects. Let's dive in and unlock the full potential of TypeScript!
"