Exploring Vue.js Composition API
By Sophia Martinez • 3/5/2025
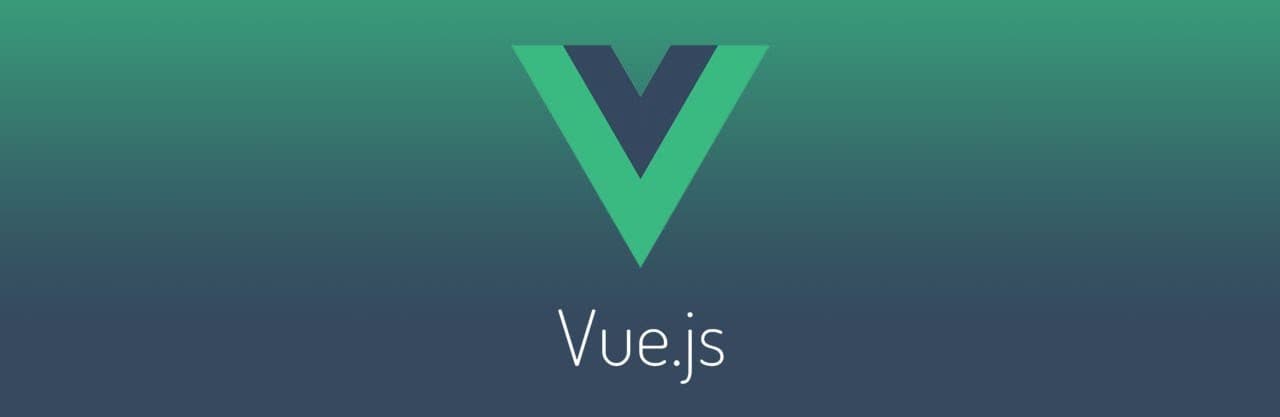
What is the Vue.js Composition API?
The Vue.js Composition API is a feature introduced in Vue 3 that provides developers with a new way to structure and reuse component logic. Unlike the Options API, which organizes code by options like data, methods, and computed properties, the Composition API organizes code by logical concerns, making it more modular and maintainable.
Key Benefits
- Improved Code Organization: Group related logic together, making components easier to read and maintain.
- Reusability: Extract reusable logic into custom composition functions.
- TypeScript Support: Better integration with TypeScript for type safety.
Getting Started
To use the Composition API, you need to import the necessary functions from Vue. Here's a simple example:
import { ref, computed } from 'vue';
export default {
setup() {
const count = ref(0);
const doubleCount = computed(() => count.value * 2);
const increment = () => {
count.value++;
};
return {
count,
doubleCount,
increment
};
}
};
Practical Example
Let's create a counter component using the Composition API:
<template>
<div>
<p>Count: {{ count }}</p>
<p>Double Count: {{ doubleCount }}</p>
<button @click=\"increment\">Increment</button>
</div>
</template>
<script>
import { ref, computed } from 'vue';
export default {
setup() {
const count = ref(0);
const doubleCount = computed(() => count.value * 2);
const increment = () => {
count.value++;
};
return {
count,
doubleCount,
increment
};
}
};
</script>
Conclusion
The Composition API is a game-changer for Vue.js developers, offering a more flexible and scalable way to manage component logic. By adopting this feature, you can improve your codebase's maintainability and reusability. Start exploring the Composition API today and unlock its full potential in your Vue.js projects!